Exploring React Phases and Lifecycle Methods
Introduction
React, a popular JavaScript library for building user interfaces, provides a set of lifecycle methods that allow developers to control and manipulate components throughout their lifecycle. These lifecycle methods offer hooks into various stages of a component’s existence, providing opportunities to initialize, update, and clean up component state and resources. In this blog post, we will explore React’s lifecycle methods, their order of execution, and how they can be leveraged to create efficient and interactive applications.
Understanding Component Lifecycle
Before diving into the specific React lifecycle methods, let’s first gain an understanding of the component lifecycle itself. In React, components undergo several stages during their existence, starting from creation, rendering, updating, and finally, unmounting. Each stage offers specific lifecycle methods that can be overridden to execute custom logic.
The React component lifecycle can be broadly divided into three phases:
- Mounting: This phase occurs when a component is being initialized and inserted into the DOM.
- Updating: This phase occurs when a component’s state or props change and requires re-rendering.
- Unmounting: This phase occurs when a component is being removed from the DOM.
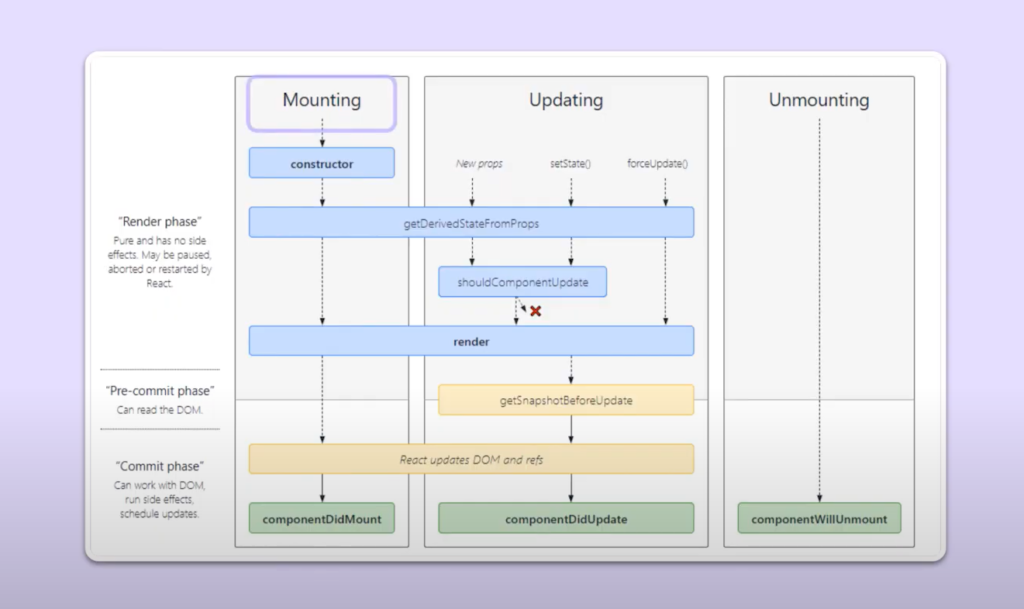
React Lifecycle Methods
- Mounting Phase:
- constructor(): This method is called when a component is first created. It is typically used for initializing state and binding event handlers.
- static getDerivedStateFromProps(): This method is invoked before rendering and allows the component to update its state based on changes in props.
- render(): This method is responsible for rendering the component’s UI.
- componentDidMount(): This method is invoked immediately after a component is mounted. It is commonly used for making API calls, setting up subscriptions, or initializing third-party libraries.
- Updating Phase:
- static getDerivedStateFromProps(): This method is also called during the updating phase, allowing the component to update its state based on changes in props.
- shouldComponentUpdate(): This method determines whether a component should re-render or not. By default, it returns true, but it can be optimized to prevent unnecessary re-renders.
- render(): Same as in the mounting phase, this method renders the component’s updated UI.
- componentDidUpdate(): This method is called immediately after an update occurs. It is often utilized for performing side effects such as updating the DOM or fetching new data based on prop or state changes.
- Unmounting Phase:
- componentWillUnmount(): This method is invoked just before a component is unmounted and destroyed. It allows for performing necessary cleanup operations such as cancelling timers, clearing subscriptions, or releasing resources.
- Other Lifecycle Methods:
- static getDerivedStateFromError(): Introduced in React 16, this method is called when a child component throws an error during rendering, allowing the parent component to capture and handle the error gracefully.
- componentDidCatch(): Also introduced in React 16, this method is used to catch and handle errors that occur during the rendering of a component.
Also read: Exploring React State And Props: A Comprehensive Guide
Also read: A Comprehensive Guide To React Components
Conclusion
React’s lifecycle methods play a vital role in controlling component behavior throughout its lifecycle. By understanding when and how to use these methods effectively, developers can optimize their components for better performance, manage state changes, and handle various scenarios.
It is important to note that React 16 introduced the concept of “Hooks,” which provide an alternative to class-based components and lifecycle methods. Hooks, such as useEffect(), useState(), useContext() etc.